图书介绍
Thinking in C++ (Second Edition) = C++编程思想 (英文版·第2版)PDF|Epub|txt|kindle电子书版本网盘下载
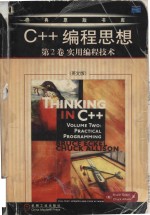
- Bruce Eckel ; Chuck Allison 著
- 出版社: China Machine Press
- ISBN:7111091622
- 出版时间:2002
- 标注页数:812页
- 文件大小:74MB
- 文件页数:835页
- 主题词:
PDF下载
下载说明
Thinking in C++ (Second Edition) = C++编程思想 (英文版·第2版)PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
Introduction1
Goals1
Chapters2
Exercises5
Exercise solutions5
Source code5
Compilers7
Language standards9
Seminars,CD-ROMs& consulting9
Errors10
About the cover10
Acknowledgements10
Ⅰ:Building Stable Systems13
1:Exception Handling15
Traditional error handling16
Throwing an exception18
Catching an exception20
The try block20
Exception handlers20
Termination and resumption22
Exception matching23
Catching any exception25
Rethrowing an exception26
Uncaught exceptions26
Cleaning up28
Resource management30
Making everything an object32
auto_ptr35
Function-level try blocks36
Standard exceptions38
Exception specifications40
Better exception specifications?45
Exception specifications and inheritance46
When not to use exception specifications47
Exception safety48
Programming with exceptions52
When to avoid exceptions52
Typical uses of exceptions54
Overhead58
Summary60
Exercises61
2:Defensive Programming63
Assertions66
A simple unit test framework70
Automated testing71
The TestSuite Framework75
Test suites79
The test framework code81
Debugging techniques87
Trace macros87
Trace file88
Finding memory leaks90
Summary96
Exercises97
Ⅱ:The Standard C++ Library101
3:Strings in Depth103
What’s in a string?104
Creating and initializing C++ strings106
Operating on strings109
Appending,inserting,and concatenating strings110
Replacing string characters112
Concatenation using nonmember overloaded operators117
Searching in strings117
Finding in reverse123
Finding first/last of a set of characters124
Removing characters from strings126
Comparing strings129
Strings and character traits134
A string application140
Summary145
Exercises146
4:Iostreams151
Why iostreams?151
Iostreams to the rescue156
Inserters and extractors156
Common usage161
Line-oriented input164
Handling stream errors165
File iostreams168
A File-Processing Example169
Open modes171
Iostream buffering173
Seeking in iostreams175
String iostreams179
Input string streams180
Output string streams182
Output stream formatting186
Format flags186
Format fields188
Width,fill,and precision190
An exhaustive example191
Manipulators194
Manipulators with arguments196
Creating manipulators199
Effectors201
Iostream examples203
Maintaining class librarysource code204
Detecting compiler errors208
A simple data logger211
Internationalization216
Wide Streams216
Locales218
Summary221
Exercises222
5:Templates in Depth227
Template parameters227
Non-type template parameters228
Default template arguments230
Template template parameters232
The typename keyword238
Using the template keyword as a hint240
Member Templates242
Function template issues245
Type deduction of function template arguments245
Function template overloading249
Taking the address of a generated function template251
Applying a function to an STL sequence255
Partial ordering of function templates259
Template specialization260
Explicit specialization261
Partial Specialization263
A practical example265
Preventing template code bloat268
Name lookup issues273
Names in templates273
Templates and friends279
Template programming idioms285
Traits285
Policies291
The curiously recurring template pattern294
Template metaprogramming297
Compile-time programming298
Expression templates308
Template compilation models315
The inclusion model315
Explicit instantiation316
The separation model319
Summary320
Exercises321
6:Generic Algorithms325
A first look325
Predicates329
Stream iterators331
Algorithm complexity333
Function objects335
Classification of function objects336
Automatic creation of function objects338
Adaptable function objects341
More function object examples343
Function pointer adaptors351
Writing your own function object adaptors358
A catalog of STL algorithms362
Support tools for example creation365
Filling and generating368
Counting370
Manipulating sequences372
Searching and replacing377
Comparing ranges385
Removing elements389
Sorting and operations on sorted ranges393
Heap operations403
Applying an operation to each element in a range405
Numeric algorithms413
General utilities417
Creating your own STL-style algorithms419
Summary420
Exercises421
7:Generic Containers429
Containers and iterators429
STL reference documentation431
A first look432
Containers of strings438
Inheriting from STL containers440
A plethora of iterators442
Iterators in reversible containers445
Iterator categories446
Predefined iterators448
The basic sequences:vector,list,deque454
Basic sequence operations454
vector457
deque465
Converting between sequences467
Checked random-access470
list471
Swapping sequences477
set479
A completely reusable tokenizer482
stack487
queue491
Priority queues496
Holding bits506
bitset〈n〉507
vector〈bool〉511
Associative containers513
Generators and fillers for associative containers518
The magic of maps521
Multimaps and duplicate keys523
Multisets527
Combining STL containers530
Cleaning up containers of pointers534
Creating your own containers536
STL extensions538
Non-STL containers540
Summary546
Exercises546
Ⅲ:Special Topics549
8:Runtime Type Identification551
Runtime casts551
The typeid operator557
Casting to intermediate levels560
void pointers561
Using RTTI with templates562
Multiple inheritance563
Sensible uses for RTTI564
A trash recycler565
Mechanism and overhead of RTTI570
Summary570
Exercises571
9:Multiple Inheritance573
Perspective573
Interface inheritance575
Implementation inheritance579
Duplicate subobjects585
Virtual base classes589
Name lookup issues599
Avoiding MI603
Extending an interface603
Summary608
Exercises609
10:Design Patterns613
The pattern concept613
Prefer composition to inheritance615
Classifying patterns615
Features,idioms,patterns616
Simplifying Idioms617
Messenger617
Collecting Parameter618
Singleton619
Variations on Singleton621
Command:choosing the operation626
Decoupling event handling with Command628
Object decoupling631
Proxy:fronting for another object632
State:changing object behavior634
Adapter636
Template Method639
Strategy:choosing the algorithm at runtime640
Chain of Responsibility:trying a sequence of strategies642
Factories:encapsulating object creation645
Polymorphic factories647
Abstract factories651
Virtual constructors654
Builder:creating complex objects660
Observer667
The “inner class” idiom671
The observer example674
Multiple dispatching679
Multiple dispatching with Visitor683
Summary687
Exercises688
11:Concurrency691
Motivation692
Concurrency in C++694
Installing ZThreads695
Defining Tasks696
Using Threads698
Creating responsive user interfaces700
Simplifying with Executors702
Yielding706
Sleeping707
Priority709
Sharing limited resources711
Ensuring the existence of objects711
Improperly accessing resources715
Controlling access719
Simplified coding with Guards721
Thread local storage724
Terminating tasks727
Preventing iostream collision727
The ornamental garden728
Terminating when blocked733
Interruption735
Cooperation between threads741
Wait and signal742
Producer-consumer relationships747
Solving threading problems with queues750
Broadcast757
Deadlock764
Summary770
Exercises773
A:Recommended Readin777
General C++777
Bruce’s books777
Chuck’s books779
In-depth C++779
Design Patterns781
B:Etc783
Index791